今さら感がありますが、最近よくGoを使っててあらためてEmacs環境を整備したのでざっくりまとめてみます。
メジャーモードの導入(go-mode)
まずGo言語のためのメジャーモードのgo-modeで入れます。
シンタックスハイライトやインデント、goコマンドをelispインタフェース通して利用できるようになります。
1 | M-x package-install go-mode |
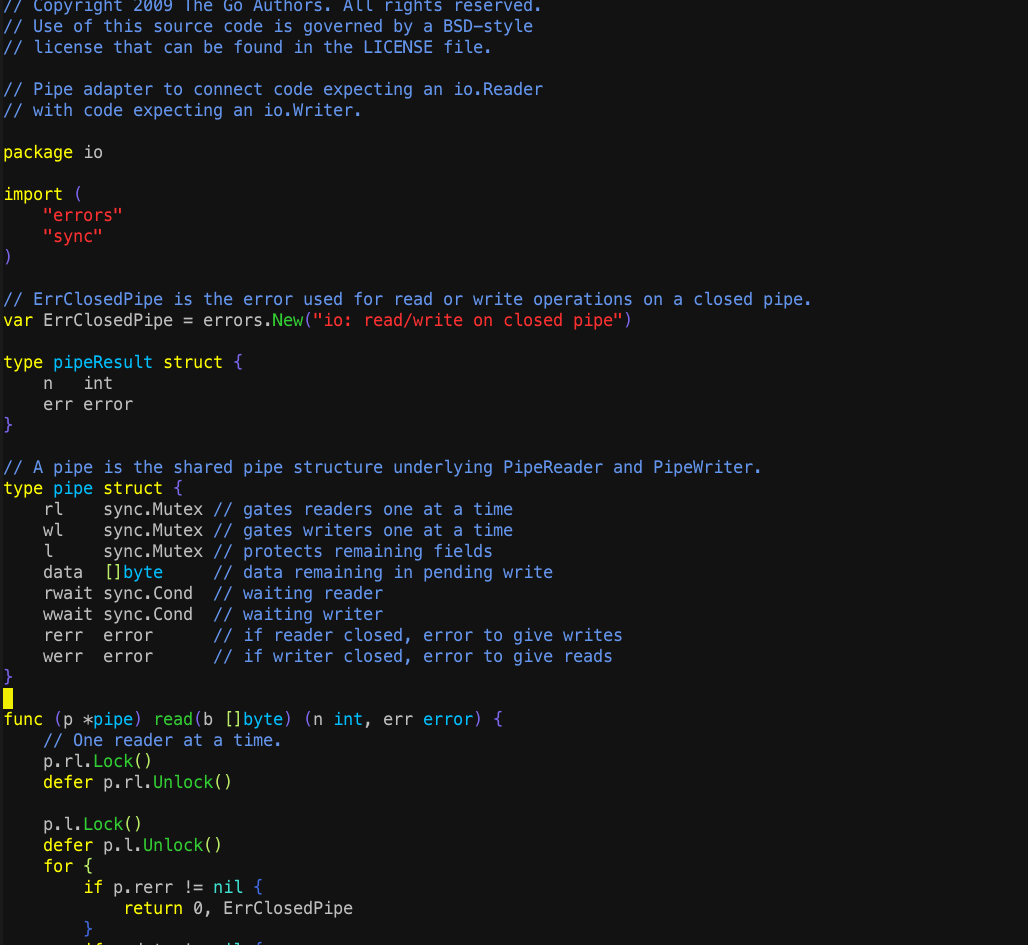
環境変数設定
go-modeやGoに関連する作業にGOPATH
が必要になるのでEmacsにも環境変数を設定します。
1 | (setenv "GOPATH" "/home/dev/go") |
タグジャンプ設定(helm-gtags)
定義元へのジャンプは godef
がよく紹介されてますが他言語の開発とタグジャンプ操作は同じインタフェースにしたいのでGnu Global + Pygments
を利用したhelm-gtags
でタグジャンプ設定します。
GOPATH直下でタグDBを作成するのですべての定義元へジャンプできるようになります。
導入方法はこの辺りを参考
https://qiita.com/yoshizow/items/9cc0236ac0249e0638ff
https://github.com/syohex/emacs-helm-gtags
http://blog.10rane.com/2014/09/17/to-reading-comprehension-of-the-source-code-by-introducing-the-helm-gtags-mode/
Go言語はまだPygmentsでサポートされていないので.ctags
に以下を追加します
1 | --langdef=Go |
コード補完(go-autocomplete)
コード補完はgocode
を利用するので事前インストールします
1 | $ go get -u github.com/nsf/gocode |
auto-complete.el
を利用したインタフェースで補完しますのでこちらをインストールして、
その後にGo言語の補完で利用するgo-autocomplete
をインストールします。
1 | (require 'go-autocomplete) |
エラーチェック(go-flymake)
エラーチェックはgo-flymakeを利用します。
入力のたびにGoのSyntaxを自動チェックして知らせてくれるようになります。
1 | (require 'go-flymake) |
ドキュメント確認
go-eldoc
カーソル位置のシンボルのドキュメントをミニバッファに表示してくれます
1 | M-x package-install go-eldoc |
パッケージ単位でドキュメントを確認
ここのやつをちょっといじってるだけです
http://syohex.hatenablog.com/entry/20130618/1371567527
helm経由でパッケージを選んでドキュメントを確認できるようになります
1 | (defvar helm-go-packages-source |
ポップアップでドキュメントを確認
go-eldoc
とほぼ同じ機能なのですが、ミニバッファだと見にくかったり、詳細がなかったり、他とイベントとカブったとき表示されないのでPopupで確認できるようにしました。
操作はリージョン選択してgodoc-popup
を実行するだけです。(popup.elが必要になります)
1 | (defun godoc-popup () |
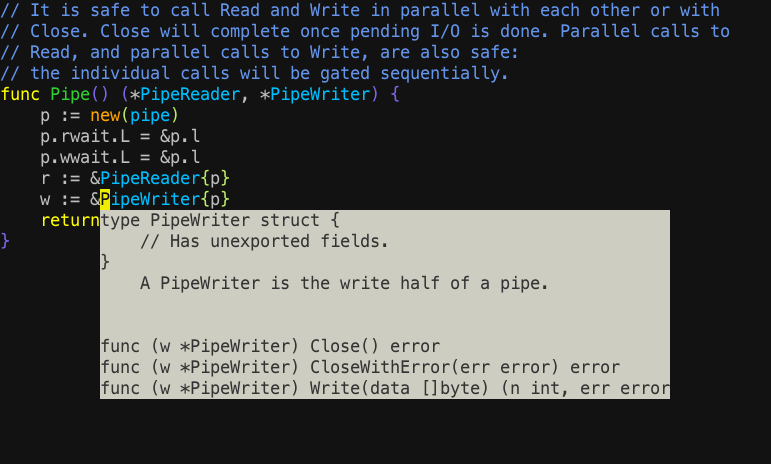
任意パッケージをdiredで開く
こちらはhelm-ghq.elで実現できたのですが、目的のパッケージをdiredで開くだけのシンプルなものがほしかったので用意しました。(事前にghqコマンドをインストールしておく必要があります)
1 | (defun helm-ghq--get-candidates () |
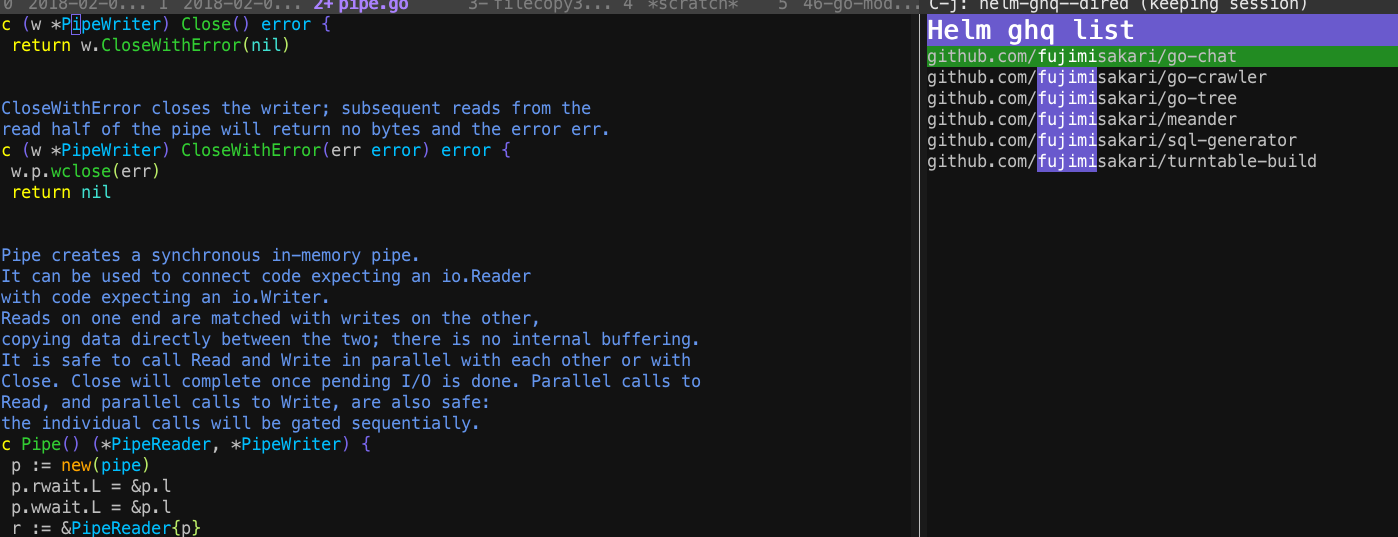
Tips
コード整形
go-modeのデフォルト機能のgofmt
を利用する。現在のバッファに対してコード整形が適用されます。
また保存時自動的にこのコマンドを実行したい場合は 次のようにhookに登録します。
1 | (add-hook 'before-save-hook 'gofmt-before-save) |
パッケージのimport
こちらもgo-modeのデフォルト機能のgo-import-add
を利用します。helm経由で選択できるので便利。
1 | go-import-add (Default: C-c C-a) |
また、使っていないimportも go-remove-unused-imports
を使うと使用していないpackageたちをimportから一括で外してくれるはずだがなぜかできない。。
コード整形とimportの整理を同時にしてくれる
goimports
はgofmtの関連ツールで標準のディストリビューションには入ってませんが
コード整形と必要に応じてインポートの宣言の挿入と削除を同時に行ってくれます。
1 | $ go get golang.org/x/tools/cmd/goimports |
godocのバッファー名は*godoc*
にする
go-mode機能のgodoc使った場合に、新たにバッファが生成されどんどん増えていくのでpopwinで無駄なバッファを生成しないようにしたいのですが
バッファー名が*godoc xxxxx*
みたいに毎回変わりpopwin設定ができないのでバッファー名は*godoc*
固定となるように関数を上書きます。
1 | (defun godoc--get-buffer (query) |
ショートカット
若干めんどうだったのでショートカットを用意しました
1 | (defun insert-go-channel-arrow () |
現在の設定
https://github.com/fujimisakari/.emacs.d/blob/master/inits/46-go-mode.el
参考サイト
http://emacs-jp.github.io/programming/golang.html
http://syohex.hatenablog.com/entry/20130618/1371567527
http://unknownplace.org/archives/golang-editing-with-emacs.html